Python Enumerate Function
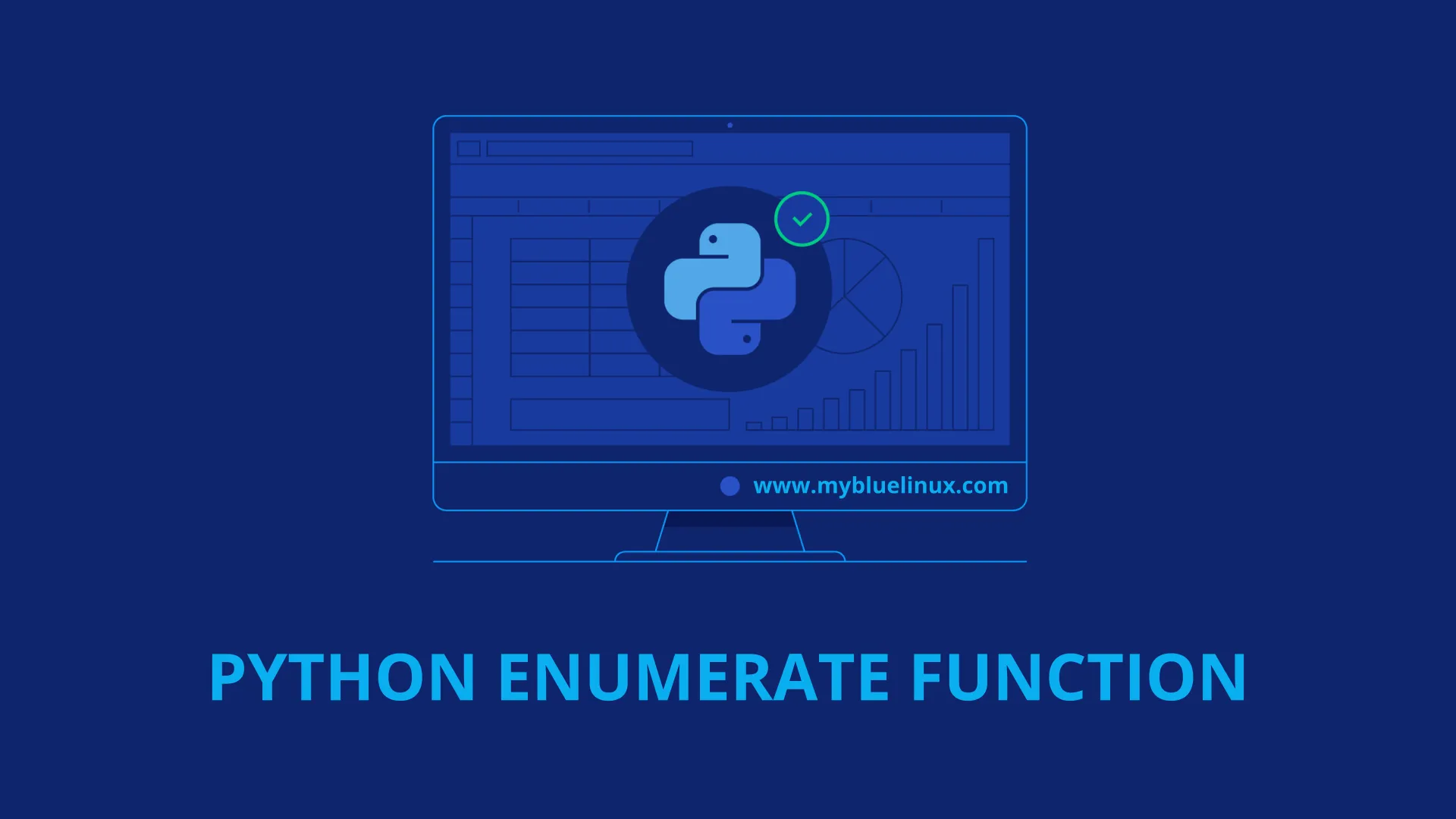
Enumerate
Enumerate is a built-in function of Python. It’s usefulness can not be summarized in a single line. Yet most of the newcomers and even some advanced programmers are unaware of it. It allows us to loop over something and have an automatic counter.
The enumerate() method adds counter to an iterable and returns it. The returned object is a enumerate object. You can convert enumerate objects to list and tuple using list() and tuple() method respectively.
Python enumerate() Syntax
enumerate(iterable, start=0)
- iterable – Required. Iterable must be a sequence, an iterator, or some other object which supports iteration.
- start – Optional. It’s the index at which enumeration shall start. If not included, enumeration starts from position 0.
The returned object is a enumerate object. You can convert enumerate objects to list and tuple using list()
and tuple()
method respectively.
How does Python enumerate() works?
Let’s explain with an example.
>>> my_list = ["one", "two", "three", "four", "five"]
>>> for i in enumerate(my_list):
... print(i)
...
(0, 'one')
(1, 'two')
(2, 'three')
(3, 'four')
(4, 'five')
>>> for i, k in enumerate(my_list):
... print(i, k)
...
0 one
1 two
2 three
3 four
4 five
>>> for i, k in enumerate(my_list, start = 10):
... print(i, k)
...
10 one
11 two
12 three
13 four
14 five
Explanation on dictionoary
>>> my_dict = {"one": "1", "two": "2", "three": "3", "four": "4", "five": "5"}
>>> my_dict.keys()
dict_keys(['four', 'two', 'one', 'five', 'three'])
>>> my_dict.values()
dict_values(['4', '2', '1', '5', '3'])
>>> my_dict.items()
dict_items([('four', '4'), ('two', '2'), ('one', '1'), ('five', '5'), ('three', '3')])
>>> for i in enumerate(my_dict):
... print(i)
...
(0, 'four')
(1, 'two')
(2, 'one')
(3, 'five')
(4, 'three')
>>> for i,k in enumerate(my_dict):
... print(i, k)
...
0 four
1 two
2 one
3 five
4 three
>>> for i,k in enumerate(my_dict.values()):
... print(i, k)
...
0 4
1 2
2 1
3 5
4 3
>>> for i,k in enumerate(my_dict.keys()):
... print(i, k)
...
0 four
1 two
2 one
3 five
4 three
>>> for i,k in enumerate(my_dict.items()):
... print(i, k)
...
0 ('four', '4')
1 ('two', '2')
2 ('one', '1')
3 ('five', '5')
4 ('three', '3')
>>> for i, (k, v) in enumerate(my_dict.items()):
... print(i, k, v)
...
0 four 4
1 two 2
2 one 1
3 five 5
4 three 3
>>> for i, (k, v) in enumerate(my_dict.items(), 1):
... print(i, k, v)
...
1 four 4
2 two 2
3 one 1
4 five 5
5 three 3