Mastering Django Class-Based Views: Django CBV
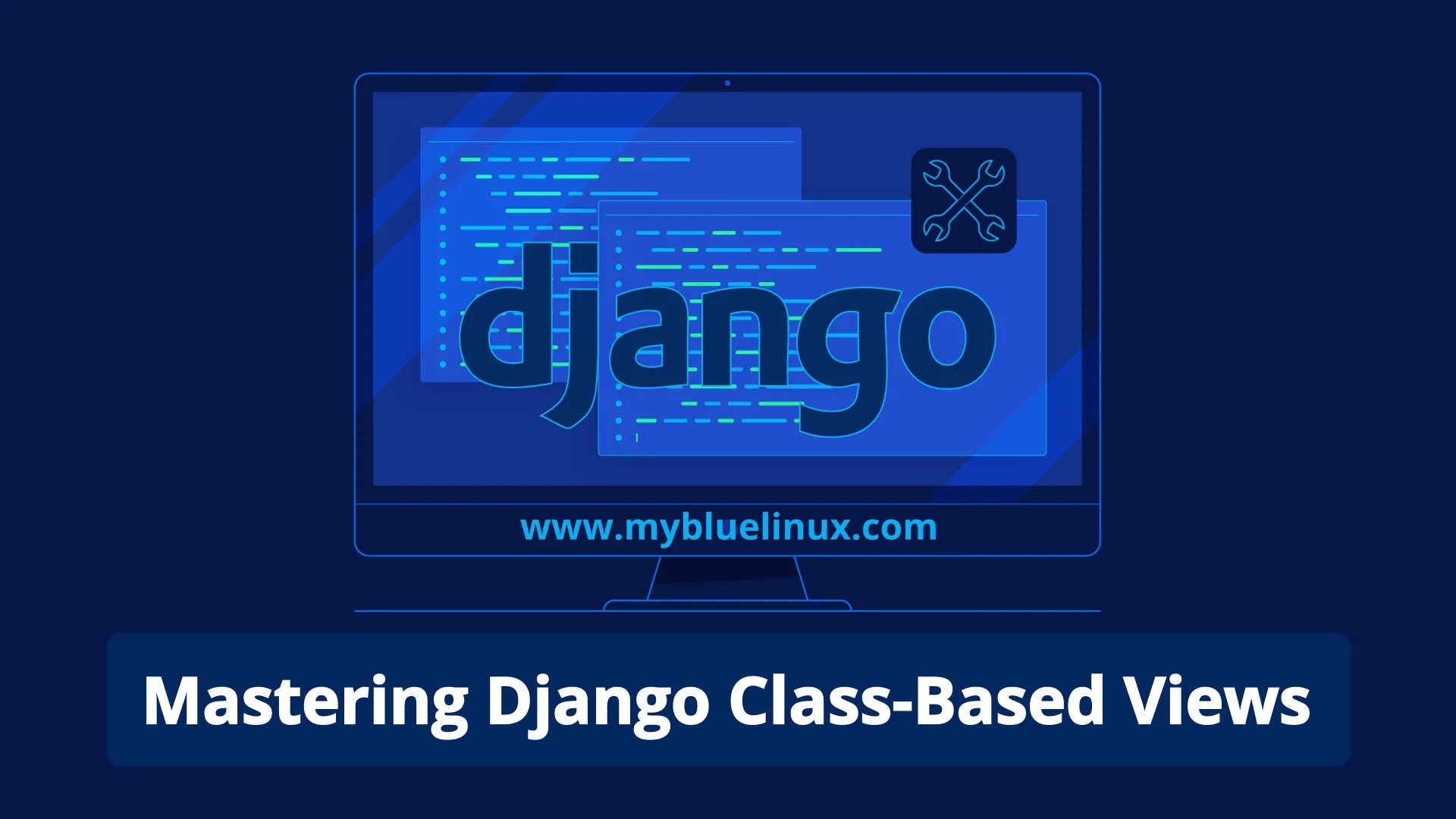
In the bustling city of Web Development, there was a street known to all Django developers, where the old, charming buildings of Function-Based Views (FBV) stood. These edifices, with their simple facades and familiar corners, had housed many developers during their early coding adventures. They were comfortable, yet each building had its own set of winding staircases and hidden rooms, making complex navigation a daily struggle for the growing population of advanced developers.
One bright morning, amidst the clatter of keystrokes, a whisper began to spread about a new architectural marvel across town — the Class-Based Views (CBV). This modern district promised a structured life with reusable components, a place where common patterns were sculpted into the very foundation, reducing the need for the repetitive, boilerplate code that often clouded the sunny skies of efficiency.
The Pivot to Elegance
Our story, dear developer, pivots here. Let us walk through the cobblestone pathways of Django views, from the all-too-familiar town of FBVs, across the bridge of understanding, to the sleek avenues of CBVs. We will embark on a journey together, leaving behind the manual labor of handling requests and responses piece by piece. Instead, we shall embrace a world where the heavy lifting is often done for us, leaving room to admire the view and focus on the unique flourishes that make our web applications truly stand out.
Chapter 1: The Journey from Functions to Classes
Once upon a time, in the early chapters of Django's own story, there was a widely traveled path named Function-Based Views (FBV). This path was well-trodden by developers carrying their requests and responses manually, crafting each view with the individual attention of an artisan. They wrote views that were procedural tales, each telling the story of a HTTP request's adventure from arrival to response.
As the tales grew more complex, with subplots of forms and side stories of HTTP methods, the path became strewn with repeated patterns. Developers would often find themselves retelling the same tales, writing the same stories over and over again, their scripts swelling with duplicate lines.
Then, from the heart of Django's development, a new chapter emerged: the era of Class-Based Views (CBV). With these, the framework offered not just a new path, but a whole new dimension. CBVs rose like grand structures from the ground, each a potential masterpiece of design patterns and reusable components.
The Architectural Renaissance
To fully appreciate the elegance of CBVs, one must first understand the object-oriented principles that underpin them. Imagine each view as a building. In the FBV district, each building is unique, constructed brick by brick for its specific purpose. However, in the land of CBVs, buildings are made using blueprints — classes that define common structures and behaviors.
These blueprints allow us to inherit and extend, to build skyscrapers upon solid foundations with minimal effort. They encapsulate behaviors in methods and properties, providing a toolkit for responding to HTTP requests. Here, get() and post() are not merely functions but are methods overridden in child classes to tell the tales of GET and POST requests with elegance and brevity.
Comparing the Constructs
Let us lay out the blueprints of FBVs and CBVs side by side, analyzing the structure of a simple “List” feature that displays articles:
Feature | Function-Based View | Class-Based View |
---|---|---|
Setup | import necessary functions | import the generic ListView |
QuerySet | define queryset inside the view functions | define queryset as a class atribute |
Response | Manually render the template with context | Automatically render the template with the get_context_data method |
Customization | add conditional logic or decorators for aditional features | extend of mix in other classes for additional features |
Reusability | extract common logic into separate functions | use inheritance to create mixins for common logic |
Readability | can become cluttered with nested logic | more organized and segmented into class methods and properties |
Consider a simple FBV that lists articles:
def article_list(request):
articles = Article.objects.all()
return render(request, 'articles/article_list.html', {'articles': articles})
Now, observe the CBV counterpart:
from django.views.generic import ListView
class ArticleListView(ListView):
model = Article
template_name = 'articles/article_list.html'
context_object_name = 'articles'
Chapter 2: The Anatomy of a Class-Based View
In the heart of Django's metropolis, where CBVs gleam like polished gems, let's enter the grand hall of the Generic View. Here, we'll don the scrubs of a code surgeon and delve into the intricate anatomy of a CBV, discovering the vital organs that keep the web's lifeblood flowing.
Dissecting the Generic View
Imagine standing in the hallowed halls of an ancient library, each book a compendium of knowledge on particular requests. The Generic View is akin to an illustrious tome, each chapter a method or attribute waiting to be invoked. Our incision begins with the as_view() method – the grand entrance leading into the heart of the CBV mansion. It breathes life into our views, transforming class definitions into callable views ready to greet incoming HTTP requests.
Next, we delicately navigate through the dispatch() method – the central atrium from which all requests are directed to the appropriate corridors, be it GET, POST, or any other HTTP method. Here, the dispatch() method ensures that each request is meticulously handed off to the correct handler.
As we delve deeper, we encounter the get_context_data() – a versatile chamber where the context is enriched with data before being presented. It's where our view gathers its thoughts, carefully selecting the information to be displayed on the web page.
Mixin Mastery
In the toolbox of a Django developer, mixins are the Army knife — compact, extendable, and immeasurably useful. Mixins are like specialized gears in a grand clock, each performing a distinct function that, when combined, create a mechanism of precise utility.
Imagine constructing your own toolbelt, selecting from mixins like LoginRequiredMixin, UserPassesTestMixin, or FormMixin. Each can be selected and attached to your view with the ease of adding a new tool to your belt, enhancing your CBVs with powerful, modular capabilities.
Overriding Methods
Our journey through the CBV anatomy would be incomplete without the craft of overriding methods. Like choosing different narrative branches in an epic tale, overriding methods in CBVs allows us to alter the destiny of our HTTP requests.
Suppose you have a ListView that needs a particular flavor of query:
from django.views.generic import ListView
class CustomArticleListView(ListView):
model = Article
template_name = 'articles/custom_article_list.html'
def get_queryset(self):
return Article.objects.filter(author=self.request.user).order_by('-publish_date')
By overriding get_queryset, we've tailored our view to present a storyline that showcases only the articles penned by our visiting user, ordered by the drama of their publication date.
Just as an artist might alter their palette to bring a new emotion to a canvas, so might a developer override get_context_data to paint additional context onto their template:
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['now'] = timezone.now()
return context
Here, we've enriched the context with the current time, perhaps to add a timely greeting to our view.
Chapter 3: Deep Dive into Advanced CBVs
Imagine a grand detective story set in the intricate world of web frameworks, where each Class-Based View is a room with secret compartments and hidden levers. It's here that our developers, turned sleuths, are tasked with unraveling the mysteries of complex ListViews and DetailViews, tailoring FormViews as meticulously as a master tailor with needle and thread, and concocting custom mixins with the precision of a gourmet chef.
Complex List and Detail Views
As we turn the pages of our casebook to the chapter on ListViews, we are faced with the enigma of pagination, filtering, and sorting. It's not just about displaying a list of objects anymore; it's about presenting a narrative that unfolds according to the reader's choices.
from django.views.generic import ListView
from django.db.models import Q
from .models import MysteryNovel
class AdvancedMysteryNovelListView(ListView):
model = MysteryNovel
paginate_by = 10
template_name = 'novels/mystery_list.html'
def get_queryset(self):
queryset = super().get_queryset()
return queryset.filter(genre='mystery').order_by('-publish_date')
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['now'] = timezone.now()
context['favorite'] = self.request.session.get('favorite_novel')
return context
In the above scene, our ListView has evolved. It's now filtering by genre, ordering by publish date, and even recalling the user's favorite novel from a past visit, stored in the session like a bookmark in a thriller.
Moving to DetailViews, the plot thickens. Here we see details of individual items, but with a twist – related data and historical context are woven into the narrative, creating a rich tapestry of information.
from django.views.generic import DetailView
from .models import Author
class AuthorDetailView(DetailView):
model = Author
template_name = 'authors/detail.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['mystery_novels'] = self.object.novels.filter(genre='mystery')
return context
Here, the DetailView not only presents the author but also a curated list of their mystery novels, allowing the reader to delve deeper into the author's world.
Building Robust Form Views
Our story now takes us to the atelier of FormViews, where forms are not just filled out but are crafted with the same attention to detail as a bespoke suit.
from django.views.generic.edit import FormView
from .forms import CustomContactForm
class CustomContactFormView(FormView):
form_class = CustomContactForm
template_name = 'contact/contact_form.html'
success_url = '/thanks/'
def form_valid(self, form):
# Process the data in form.cleaned_data
return super().form_valid(form)
In the above narrative, every stitch of the FormView is placed with intention. Upon submission, the form's data is not merely sent but is processed, each field's data woven into the backend like fine thread.
Implementing Custom Mixins
Finally, we approach the climactic scene — creating custom mixins. Like a chef carefully selecting ingredients to create the perfect flavor profile, we combine properties and methods to craft a mixin that adds the desired functionality to our views.
from django.contrib.auth.mixins import UserPassesTestMixin
class AuthorOnlyMixin(UserPassesTestMixin):
def test_func(self):
return self.request.user.is_author
def handle_no_permission(self):
return render(self.request, 'errors/not_author.html')
With the AuthorOnlyMixin, we've concocted a special ingredient that ensures only authors can access certain views, redirecting mere mortals to a polite refusal page.
Chapter 4: Unraveling the Mysteries of the Django View Middleware
In the sprawling metropolis of Django, middleware is the unseen yet ever-vigilant security system, a network of checkpoints and filters that scrutinizes the flow of requests and responses. When it comes to Class-Based Views, this system becomes even more nuanced, a subtle dance of permissions and protocols that occurs behind the scenes of every HTTP request.
Middleware and CBVs: The High-Tech Security System
Envision a Class-Based View as a skyscraper in the city of Django, sleek and modern, towering with confidence. Middleware acts as the sophisticated security detail of this structure. Each piece of middleware is a checkpoint, the guards and systems that vet every visitor — every request — before they can interact with the inner sanctum, the view itself.
As requests enter the building lobby, they pass through metal detectors and scanners — middlewares such as SecurityMiddleware and SessionMiddleware. These ensure that the request is free of potential threats and carries the proper credentials.
When it comes to CBVs, the middleware security is even more advanced. It's not just about scanning what comes in; it's also about preparing the CBV for what it needs to do. For instance, AuthenticationMiddleware ensures that our CBV knows who is walking through its corridors, allowing the view to tailor the experience to the visitor's access level.
Practical Tips: Insider Secrets for Middleware in CBVs
For those with the right clearance, the secrets of integrating middleware with CBVs can unlock new levels of efficiency and security. Here are some insider tips to elevate your CBV game:
- Middleware Ordering Matters
- Just like the order of operations in a complex algorithm, the sequence in which middleware is applied is critical. Ensure that AuthenticationMiddleware precedes MessageMiddleware so that request.user is available for messages.
- Use Middleware to Decorate CBVs
- Sometimes, you want a specific piece of middleware to apply to just one CBV, not the whole project. You can use middleware as decorators atop your view classes for this precision.
from django.utils.decorators import method_decorator from django.views.decorators.csrf import csrf_protect from django.views.generic import View @method_decorator(csrf_protect, name='dispatch') class SecureView(View): # Your CBV code here
- Custom Middleware for CBVs
- Crafting your own middleware can give you pinpoint control over the behavior of your views. For instance, you might create a TimingMiddleware that logs how long a CBV takes to process a request, giving you insights into performance bottlenecks.
class TimingMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): start = time.time() response = self.get_response(request) duration = time.time() - start print(f"View Response Time: {duration:.2f}s") return response
- Middleware for Multi-Tenancy
- In a scenario where your CBV must serve different data sets depending on the client (multi-tenancy), a custom middleware can set up the request for the CBV, filtering data based on the client's domain.
class TenantMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): tenant_domain = request.get_host().split('.')[0] request.tenant = Tenant.objects.get(domain_prefix=tenant_domain) return self.get_response(request)
Chapter 5: Real-World Applications
As we delve into this chapter, we find our seasoned developers gathered around a table cluttered with notes and diagrams. They're facing a formidable challenge: a real-world problem that demands not just code, but cunning and foresight. It's a detective story, and Class-Based Views (CBVs) are the magnifying glass through which they'll examine the clues.
Case Study: The Mystery of the Inefficient Dashboard
Our story unfolds with The Inefficient Dashboard, a system so slow and unwieldy that users are beginning to turn away. It's a complex interface, aggregating data from numerous sources, and presenting them in real-time. The developers' mission: to refactor this labyrinthine codebase using CBVs, to unearth the bottlenecks and reimagine the data flow.
from django.views.generic import TemplateView
from .services import gather_user_data, gather_system_stats
class DashboardView(TemplateView):
template_name = 'dashboard.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['user_data'] = gather_user_data(self.request.user)
context['system_stats'] = gather_system_stats()
return context
In the snippet above, our detectives have started by breaking down the monolithic function into discrete services, each responsible for a piece of the puzzle. The CBV DashboardView calls upon these services, each a clue to untangling the spaghetti of the former code.
Performance Considerations: The Strategist's Campaign
But the plot thickens: high performance is not just about clean code — it's about strategy. Like generals at war, the developers must consider their tactics: caching strategies, query optimizations, and the art of asynchronous code.
- Caching
- The developers implement strategic caching, storing the results of expensive queries like treasure maps, to be used when the same request comes knocking.
from django.core.cache import cache
from .models import ExpensiveDataQuery
class CachedDetailView(DetailView):
model = ExpensiveDataQuery
template_name = 'details.html'
def get_object(self, queryset=None):
obj = cache.get(f'expensive_query_{self.kwargs["pk"]}')
if not obj:
obj = super().get_object(queryset=queryset)
cache.set(f'expensive_query_{self.kwargs["pk"]}', obj, timeout=3600)
return obj
- Query Optimization
- Each query is scrutinized, optimized like a codebreaker parsing an encrypted message, using Django's select_related and prefetch_related to reduce database hits.
from django.views.generic.list import ListView
from .models import Book
class OptimizedBookListView(ListView):
model = Book
template_name = 'book_list.html'
def get_queryset(self):
# Prefetch related data to avoid N+1 queries
return Book.objects.prefetch_related('author').all()
- Asynchronous Code
- To handle I/O-bound operations without keeping users waiting, they turn to Django's support for asynchronous views, a sleight of hand that keeps the show running while the magic happens backstage.
from django.views.generic import TemplateView
from asgiref.sync import sync_to_async
from .heavy_lifting import perform_heavy_lifting
class AsyncHeavyLiftingView(TemplateView):
template_name = 'async_view.html'
async def get_context_data(self, **kwargs):
context = await super().get_context_data(**kwargs)
heavy_lifting_result = await sync_to_async(perform_heavy_lifting, thread_sensitive=True)()
context['heavy_lifting_result'] = heavy_lifting_result
return context
Chapter 6: CBVs in the Wild — Beyond the Django ORM
This chapter takes us beyond the familiar trails of the Django ORM into the wilds where third-party services roam, and where the asynchronous awaits to be harnessed. Our seasoned developers stand on the edge, peering into a landscape where Class-Based Views must interact with external APIs and handle operations without the safety net of Django's ORM.
Integration with Other Services: The Crossroads of Collaboration
The journey begins at a crossroads, a place where Django's CBVs must shake hands with external services. They are tasked with incorporating an external search engine into their project, and the CBVs must adapt to communicate effectively with this foreign entity.
from django.views.generic import ListView
import requests
class ExternalSearchView(ListView):
template_name = 'search_results.html'
def get_queryset(self):
response = requests.get(
'https://api.externalsearch.com/items',
params={'query': self.request.GET.get('q')}
)
response.raise_for_status()
return response.json()['items']
In the snippet above, ExternalSearchView steps out of the ORM's comfort zone, sending out an emissary—in the form of an HTTP GET request—to gather data from an external search API. The CBV then translates the foreign response into a format that its templates can understand, bridging the gap between Django and the rest of the world.
Async Views: The New Frontier
The developers next turn their gaze to the horizon, where Django's asynchronous capabilities shimmer in the distance. This is the new frontier, where CBVs must perform without blocking the passage of requests, keeping the flow smooth and uninterrupted.
from django.http import JsonResponse
from django.views.generic import View
from asgiref.sync import async_to_sync
from .tasks import async_external_api_call
class AsyncExternalAPIView(View):
def get(self, request, *args, **kwargs):
query = request.GET.get('q')
# Asynchronous call to an external API
data = async_to_sync(async_external_api_call)(query)
return JsonResponse(data)
In the example, AsyncExternalAPIView demonstrates how to send an asynchronous call to an external service. It's a quest for data that doesn't block the paths of other requests, letting them pass freely while it awaits the return of its asynchronous knights.
As our developers conclude their journey with CBVs, they have not only navigated through the realms of third-party services but also blazed trails with asynchronous views. They have ventured into territories where many fear to tread, wielding CBVs as their compass and Django's asynchronous features as their map.
Chapter 7: The Art of Debugging CBVs
Our journey through the landscape of Django's Class-Based Views reaches an intriguing chapter — the art of debugging. It's a craft as old as code itself, a skill that turns developers into detectives, their screens into canvases of possibilities where every line could hide a clue. This chapter is the compendium of their most thrilling cases, the tales of bugs so elusive they became legends, and the arsenal of tools that turned bug hunts into masterclasses of deduction.
Debugging Stories: The Case of the Silent Failure
Once upon a time, in a codebase not too distant, there was a CBV so convoluted, it failed silently in the night. No trace, no error, just a blank screen staring back at the user. The developers gathered, their eyes narrowed in concentration as they pored over logs and traces. They added custom logging in the view's dispatch method, sprinkling print statements like breadcrumbs:
import logging
class MyComplexListView(ListView):
# ...
def dispatch(self, request, *args, **kwargs):
logging.debug(f"Dispatching {self.__class__.__name__}")
return super().dispatch(request, *args, **kwargs)
With logs as their lanterns, they traced the execution through the dark forest of code until they found the rogue code hiding in a get_context_data override, failing silently because of an unhandled exception. The bug, once a shadow, became as clear as day.
Advanced Debugging Tools: The Detective's Gadgets
But tales of logging were not enough. The developers needed tools, gadgets that would make their quest less daunting. They turned to Django Debug Toolbar, a veritable Army knife for debugging, attaching it to their local setup to unveil the mysteries of SQL queries, template rendering, and cache behavior.
For times when deeper introspection was required, they wielded the power of pdb, Python's debugger, embedding it into their CBV to freeze time and space:
from django.views.generic import View
import pdb
class MyIntricateView(View):
def get(self, request, *args, **kwargs):
pdb.set_trace()
# The world stops here, waiting for the developer's command
return super().get(request, *args, **kwargs)
And for the bravest, who sought to understand the very soul of their CBVs, they used django-extensions runserver_plus and werkzeug, turning their servers into gateways of introspection, allowing them to travel through code execution in real-time, inspecting variables and stack traces as if they were relics of ancient code.
Chapter 8: Testing Your CBVs
In the grand saga of our Django development, there comes a chapter where heroes don't just forge their code in the fires of creation but also armor it against the future — a chapter dedicated to the art of testing Class-Based Views.
Crafting a Testing Strategy: The Tale of the Bulletproof Vest
The story unfolds in a digital realm where bugs and unexpected behaviors are the foes, and tests are the armor. Our developers are like meticulous armorers, crafting each piece of their testing suit with care, ensuring it fits perfectly and protects against all manner of attacks.
They begin by penning a tale of TestCase scenarios, where each scenario is a dragon to be slain:
from django.test import TestCase
from django.urls import reverse
class MyViewTest(TestCase):
def test_get(self):
response = self.client.get(reverse('my_view'))
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'my_template.html')
With this simple story, our developers have a trustworthy shield, a test that ensures their view responds as expected and renders the right scroll — or template, in the language of mortals.
Mocking and Patching in CBVs: The Special Effects of the Code World
As our developers venture further, they encounter scenarios where real-world entities cannot be called upon — external APIs, database calls, time itself. Here, they turn to the art of illusions, to the craft of mocking and patching, much like the special effects wizards in the film industry who conjure dragons out of smoke and bravery out of light.
from unittest.mock import patch
from django.test import TestCase
from .views import ExternalAPIView
class ExternalAPITest(TestCase):
@patch('myapp.views.requests.get')
def test_external_api_view(self, mock_get):
mock_get.return_value.json.return_value = {'key': 'value'}
response = self.client.get(reverse('external_api'))
self.assertEqual(response.status_code, 200)
self.assertEqual(response.context['data'], {'key': 'value'})
In the script above, patch is the special effects team, creating a controlled environment where external calls are mere illusions, ensuring the CBV can be tested without the unpredictability of external dependencies.
As the chapter draws to a close, our developers stand back and admire their handiwork. Their CBVs, once vulnerable, are now clad in an armor of tests. They understand that each test is a story in itself, a scenario played out against the backdrop of their code, ensuring that when the real trials come, their code stands tall and unbroken.
Chapter 9: Customizing the Django Admin with CBVs
In the annals of Django development, the admin interface stands as the central stronghold, a powerful bastion that oversees the realm of models and data. And yet, there are times when the standard defenses of this fortress must be tailored, fortified by the hands of code-smiths who mold Class-Based Views into armor fit for the kings and queens of admin land.
Tales from the Admin Interface: The Armorer's Craft
Our developers, skilled artisans in the craft of code, gather around the anvils of their IDEs, intent on customizing the Django admin. They share tales of extending ModelAdmin with custom views, of adding buttons and actions that make the interface not just functional but legendary:
from django.contrib import admin
from django.http import HttpResponseRedirect
from django.urls import path
from .models import Hero
@admin.register(Hero)
class HeroAdmin(admin.ModelAdmin):
change_list_template = "admin/heroes_change_list.html"
def get_urls(self):
urls = super().get_urls()
custom_urls = [
path('armor-up/', self.admin_site.admin_view(self.armor_up), name='armor-up'),
]
return custom_urls + urls
def armor_up(self, request):
# The method that will be called when the 'Armor Up' button is clicked
# Code to armor up the heroes goes here
self.message_user(request, "The heroes have been armored up!")
return HttpResponseRedirect(".")
In this narrative, the armor_up method is a powerful spell, cast to bestow additional protection upon the data-heroes that dwell within the admin interface, a custom action invoked with a click as if drawing a sword for battle.
Permission Handling: The Enchantments of Access
But what is a stronghold without its guards, its protocols that determine who may enter and who must remain outside its walls? Our developers understand that in the admin interface, permissions are these very enchantments, intricate and powerful:
from django.contrib import admin
from django.contrib.auth.mixins import PermissionRequiredMixin
class CustomAdminView(PermissionRequiredMixin, admin.ModelAdmin):
# Set the necessary permission
permission_required = ('myapp.change_hero',)
def has_change_permission(self, request, obj=None):
# Custom permission logic
if request.user.is_superuser:
return True
return super().has_change_permission(request, obj=obj)
In the script of their story, permissions are woven into the CBVs with the care of a master enchanter, ensuring that only those with the right incantations — or user permissions — can alter the fabric of the admin interface.
Chapter 10: Best Practices and Design Patterns
In every craftsman's tale, there's a chapter where the wisdom of ages is distilled into lessons for the future. For those who wield the power of Django's Class-Based Views, such wisdom is found in the best practices and design patterns, the sacred scrolls that separate the master from the apprentice.
Patterns and Anti-Patterns: The Cartographer's Guide to CBVs
Our developers are like seasoned cartographers, mapping the landscape of CBVs with an unerring eye for routes that lead to elegance and those that descend into the mire. They discuss design patterns — the well-trodden paths that lead to success:
# The Template Method Pattern in a CBV
from django.views.generic import TemplateView
class BaseView(TemplateView):
template_name = 'my_base_template.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['universal_data'] = self.get_universal_data()
return context
def get_universal_data(self):
# Method to be overridden with specific data in subclasses
return {"site_name": "MyDjangoSite"}
In this narrative, design patterns like the Template Method are depicted as foundational stones, upon which the mighty temples of CBVs are built, allowing common behaviors to be defined in a base class, while specific behaviors are etched into its descendants.
Conversely, they warn of anti-patterns — the forbidden spells that lead to a CBV's ruin:
# An example of an anti-pattern: Logic Overload in a CBV
from django.views.generic import ListView
class MyOverloadedView(ListView):
# ...
def get_queryset(self):
# An overloaded method that does too much, making it hard to follow and maintain.
# ...
pass
def get_context_data(self, **kwargs):
# An overloaded method that is trying to handle too many contexts.
# ...
pass
In this lore, anti-patterns are akin to quicksand, seemingly easy paths that trap developers in maintenance nightmares, where methods become so overloaded with logic that they lose clarity and purpose.
Maintainability and Extensibility: The Master Craftsmanship in CBVs
The sages of our story then turn to the twin pillars of CBV architecture: maintainability and extensibility. They recount tales of CBVs designed with foresight, where future changes are but a minor enchantment away:
# Extensibility in a CBV
class MyBaseView(TemplateView):
extra_context = None
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
if self.extra_context is not None:
context.update(self.extra_context)
return context
# This design allows subclasses to easily add to the context.
The wisdom imparted here is akin to the ancient art of building with expansion in mind, where new chambers can be added to the edifice without disturbing the structure's integrity.
As the last words of this chapter are inscribed onto parchment, our developers — now turned philosophers — reflect on the balance struck between robustness and simplicity, between a CBV that performs its duty and one that invites the fates of complexity and decay.
Conclusion: The Master's Codex
As we draw the curtains on this grand tapestry of Django's Class-Based Views, we take a moment to tread back through the chapters of our odyssey — a journey through the very heart of Django's elegance and power. We ventured from the familiar lands of function-based views and stepped into the vast kingdom of CBVs, uncovering the arcane knowledge that lies within.
Recap of the Journey: The Path of Enlightenment
From the simplest of ListViews to the intricate dance of Mixins and Middleware, we've traversed the landscape of CBVs with the tenacity of explorers discovering new territories. We've encountered the enigmas of advanced features, the storytelling of debugging sagas, and the strategic craftsmanship of testing. We've armored the admin interface with bespoke enhancements and etched the lore of best practices into our codex.
Our scripts have grown rich with the code of ages, and our scenarios have been as varied and real as the stars that guide the traveler's path.
Final Thoughts: The Mentor's Parting Wisdom
As your mentor, I stand at the gates of this journey's end, offering these final pearls of wisdom: Let the principles of simplicity and reusability be your guiding stars. May you build with foresight, ever-conscious of the balance between the robust and the refined. And as you forge ahead, let your code tell a story, one that future developers will read with admiration and clarity.
Sources