Linux Command Line Tips and Tricks
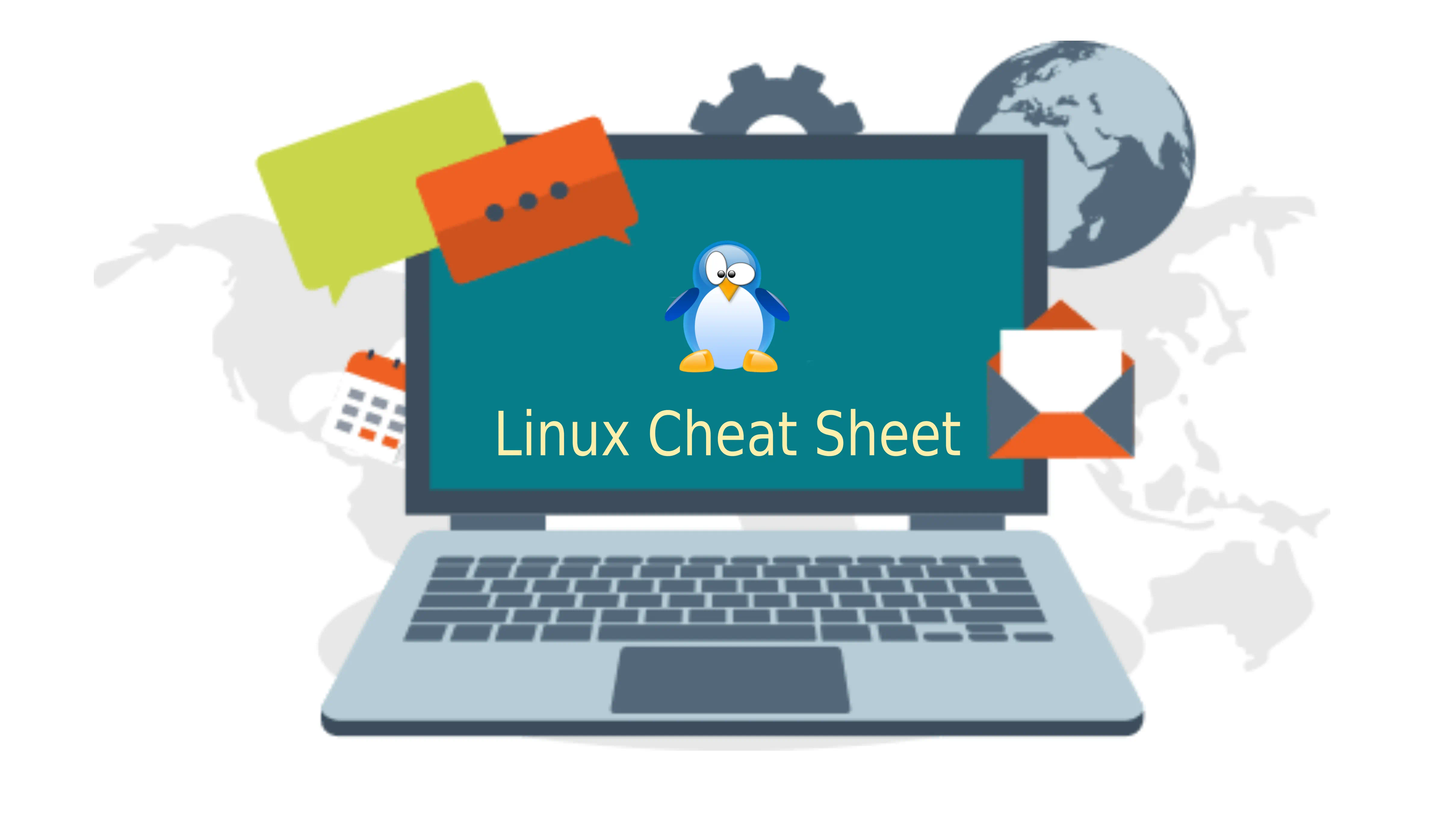
Text Editing
For mostly all examples we use sed utility with substitution command. The syntax is pretty simple:
sed -i 's/SEARCH-WORD/REPLACMENT-WORD/gI' input
# or for regular expressions:
sed -i 's/SEARCH-REGEXP/REPLACMENT-WORD/gi' input
- The
-i
option edit and update text file in place. For piped input from another utility it is not necessary - The
/
are delimiters between words or regexp. - sed -i 's/SEARCH-REGEXP/REPLACMENT-WORD/g
I
' - I flag make search case insensitive - sed -i 's/SEARCH-REGEXP/REPLACMENT-WORD/
g
I' - for text files input use g flag to make search global across the whole file
print first N chars
We want print first N chars. For example, print first 6 chars from every line of our example file:
cat example.txt | sed 's/^\(.\{1,6\}\).*/\1/'
France
Englan
Japan:
USA:Wa
Τηεοδ2
- sed 's/
^
(.{1,6}).*/\1/' - a regular expression indicating the beginning of a line - sed 's/^
\(.\{1,6\}\)
.*/\1/' - regexp for capture minimal first and max any 6 chars and remember - sed 's/^(.{1,6})
.*
/\1/' - mark all remaining characters - sed 's/^(.{1,6}).*/
\1
/' - substitute all whole line with remembered chars from step 2
print chars from N to M
~] cat example.txt
France:Paris:euro
England:London:pound sterling
Japan:Tokio:yen
USA:Washington:dollar
Τηεοδ29:Ελλάδα
We need print chars from position 3 to 8 - we need don't caputre first 2 chars and than capture next 6 chars. For our example:
# capture chars from position 3 to 8:
X = 3
Y = 8
# new variables for sed command:
N = X -1
M = Y - N
# sed command:
sed 's/^.\{M\}\(.\{1,N\}\).*/\1/'
~] cat example.txt | sed 's/^.\{2\}\(.\{1,6\}\).*/\1/'
ance:P
gland:
pan:To
A:Wash
εοδ29:
sed 's/^.\{2\}\(.\{1,6\}\).*/\1/'
- print chars from position 3 to 8- sed '
s
/^.{2}(.{1,6}).*/\1/' - command for substitution text in sed - sed 's/
^.\{2\}
(.{1,6}).*/\1/' - capture any first 2 chars (and don't remember) - sed 's/^.{2}
\(.\{1,6\}\).*
/\1/' - capture next 1-6 chars (minimal one and max exactly 6 chars) after two chars from step 1 and remember them, all chars between()
are memorized - sed 's/^.{2}(.{1,6})
.*
/\1/' - capture any possible others chars after what we remember - sed 's/^.{2}(.{1,6}).*/
\1
/' - delete all captured chars and substitute it with first capture group
- sed '
print chars from N to M for column
We need print chars from position 3 to position 6
~] cat example.txt
France:Paris:euro
England:London:pound sterling
Japan:Tokio:yen
USA:Washington:dollar
Τηεοδ29:Ελλάδα
~] cat example.txt | awk 'BEGIN { FS=":" } { print $2 }' | sed 's/^.\{2\}\(.\{1,4\}\).*/\1/'
ris
ndon
kio
shin
λάδα
- awk '
BEGIN { FS=":" }
{ print $2 }' - set input field separator variable to ":" char - awk 'BEGIN { FS=":" }
{ print $2 }
' - print only second column sed -e 's/^.\{2\}\(.\{1,4\}\).*/\1/'
- explanation is described in example above
Note:
When use a regexp for capture exactly 4 chars - {4}
, we get a mismatch. That's why we have to use regexp for capture minimal 1 chars and maximum 4 chars.
~] cat example.txt | awk 'BEGIN { FS=":" } { print $2 }' | sed 's/^.\{2\}\(.\{4\}\).*/\1/'
Paris
ndon
Tokio
shin
λάδα
append some text to every line
We need append comment char "#" to every line:
~] cat example.txt | sed 's/^/#/'
#France:Paris:euro
#England:London:pound sterling
#Japan:Tokio:yen
#USA:Washington:dollar
#Τηεοδ29:Ελλάδα
- sed '
s
/^/#/' - command for substitution text in sed - sed 's/
^
/#/' - regulax expression matched start of line - sed 's/^/
#
/' - substitute start of line with # char