How to Properly Add JavaScripts and Styles in WordPress
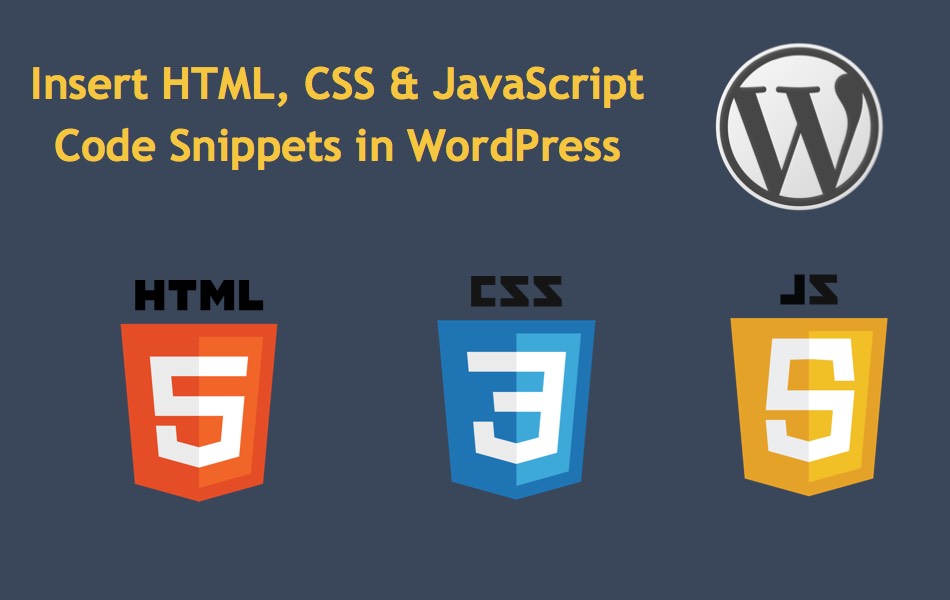
How to Properly Add JavaScripts and Styles in WordPress
Do you want to learn how to properly add JavaScripts and CSS stylesheets in WordPress? Many DIY users often make the mistake of directly calling their scripts and stylesheets in plugins and themes. In this article, we will show you how to properly add JavaScripts and stylesheet in WordPress. This will be particularly useful for those who are just starting to learn WordPress theme and plugin development.
Often times, adding custom JavaScript for analytics tracking is the first type of custom code a WordPress user has to implement. Normally the documentation that you are given with these codes are written as if your site is just a bunch of HTML files. But that’s not how WordPress works. The HTML of your site is dynamically generated.
Ways To Add Custom JavaScript To Your Site.
Here is a quick list of options for adding JavaScript to your WordPress site. They all will work, but some are better than others. I’ll go over each in detail in this article:
- Load a separate JavaScript file using WordPress’ script loader
- Use the wp_footer or wp_head hooks to add the script inline
- Use a plugin to add header or footer scripts
- Modify your theme to include the script (bad idea)
- Use the WordPress post editor (really bad idea not worth discussing)
Adding JavaScript Inline With WordPress Hooks
For small bits of code it doesn’t make sense to use a separate JavaScript file, that would require and therefore extra HTTP request when loading the page. What we need now is inline javascript code add directly to HEAD section of our html file like this:
<head>
...
...
Our code here
...
...
</head>
You can add this code to either your functions.php
file in your child theme or create it as a plugin. So, open your theme or child theme functions.php
file and add this code to the end of file:
<?php
/**
Add custom text to <head></head> header
*/
add_action( 'wp_head', 'my_header_scripts' );
function my_header_scripts(){
?>
<script>alert( 'HELLO WORLD' ); </script>
<?php
}
A footer script plugin would be almost identical, except you would use the wp_footer action.
<?php
/**
How add script to foote
*/
add_action( 'wp_footer', 'my_footer_scripts' );
function my_footer_scripts(){
?>
<script>alert( 'Hello World' ); </script>
<?php
}
How To Include A JavaScript File In WordPress The Right Way
WordPress has a system for loading JavaScript and CSS files. For JavaScript files, you can use the function wp_enqueue_scripts , inside of a callback function hooked to the wp_enqueue_script action to load a file. For large amounts of JavaScript this is a good idea.
header.php
or footer.php
but this is bad practice. The wp_enqueue_script
system manages the order scripts are loaded, scripts they are dependent on and dynamically generates the URL.
Here is skeleton what we need:
function wp_adding_scripts()
{
// Deregister the included library
wp_deregister_script( 'jquery' );
// Register the library again from Google's CDN
wp_register_script( 'jquery', 'http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js', array(), null, false );
// Register the script like this for a plugin:
wp_register_script( 'custom-script', plugins_url( '/js/custom-script.js', __FILE__ ), array( 'jquery' ) );
// or
// Register the script like this for a theme:
wp_register_script( 'custom-script', get_template_directory_uri() . '/js/custom-script.js', array( 'jquery' ) );
// or
// Register the script like this for a your child theme:
wp_register_script( 'custom-script', get_stylesheet_directory_uri() . '/js/custom-script.js', array( 'jquery' ) );
// For either a plugin or a theme, you can then enqueue the script:
wp_enqueue_script( 'custom-script' );
}
add_action( 'wp_enqueue_scripts', 'wp_adding_scripts()' );
Explanation
We started by registering our script through the wp_register_script() function. This function accepts 5 parameters:
$handle
– (string) (Required) Name of the script. Should be unique.$src
– (string) (Required) Full URL of the script, or path of the script relative to the WordPress root directory.$deps
– (array) (Optional) An array of registered script handles this script depends on. Default value: array()$ver
– (string|bool|null) (Optional) String specifying script version number, if it has one, which is added to the URL as a query string for cache busting purposes. If version is set to false, a version number is automatically added equal to current installed WordPress version. If set to null, no version is added. Default value: false$in_footer
– (bool) (Optional) Whether to enqueue the script before