Python Dictionaries and JSON
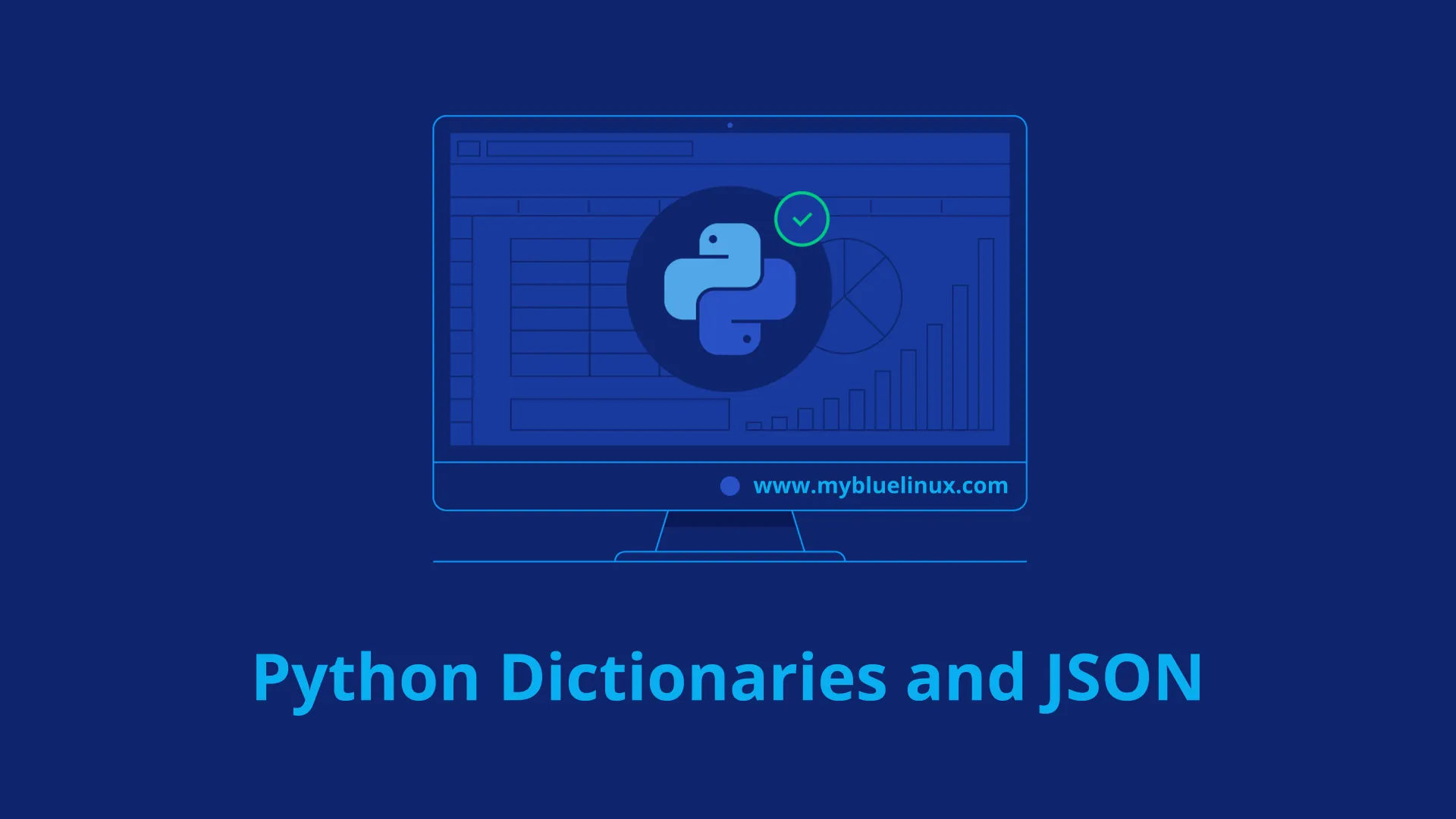
This week we will have a quick look at the use of python dictionaries and the JSON data format. After reading this post, you should have a basic understanding how to work with JSON data and dictionaries in python. I'll choose this topic because of some future posts about the work with python and APIs, where a basic understanding of the data format JSON is helpful.
What is a python dictionary?
A python dictionary is a list that is indexed by key values. The key value can be any immutable data type, strings are used in most cases. The dictionary data structure is also often called "associative arrays" in other programming languages.
A dictionary can be created using the function dict(). To access a value in a python dictionary, you use the square brackets after the variable name, as the following example describes:
>>> my_dictionary = { 'key': 'value'}
>>> print(my_dictionary["key"])
value
If the key is not found in the dictionary, an exception is thrown. To get a list of all defined keys in a dictionary, you can use the method .keys() on the dictionary object. You can also create python dictionaries using curly braces, as described in the following example:
my_dict = {
"key 1": "value 1",
"key 2": "value 2"
}
my_dict.keys()
dict_keys(['key 2', 'key 1'])
You can also add lists and nested dictionaries to the data structure and access it using multiple square brackets. The use of python dictionaries is straightforward and makes from my perspective the code more readable in many cases. If you like to know more about the python dictionary data structure, you can find further information in the official python documentation .
What is JSON?
JSON stands for JavaScript Object notation and is an open standard human readable data format. It is defined in RFC 7159
. Many APIs work with this format to provide and receive data.
Popular alternatives to JSON are YAML
and XML
. The Wikipedia article about JSON
provides a very good overview and explanation, therefore I will just summarize the basic formatting elements.
An empty JSON file simply contains two curly braces {}. The following example describes some basic value definitions. Multiple value statements in JSON are separated by a comma sign.
{
"text key": "string",
"integer key": 123,
"boolean key": true,
"simple list": [
"value 1",
"value 2"
],
"dict": {
"element 1": "value 1",
"element 2": "value 2"
},
"dict list": [
{
"key 1.1": "value 1.1",
"key 1.2": "value 1.2"
},
{
"key 2.1": "value 2.1",
"key 2.2": "value 2.2"
}
]
}
Work with dictionaries and JSON data in python
To work with JSON formatted data in python, we will use the integrated python json module . We can use this module to load any JSON formatted data from a string or a file, as the following code example describes:
import json
# you can also use the open function to read the content of a JSON file to a string
json_data = """ {
"key 1": "value 1",
"key 2": "value 2",
"decimal": 10,
"boolean": true,
"list": [1, 2, 3],
"dictionary": {
"child key 1": "child value 1",
"child key 2": "child value 2"
}
}"""
my_dict = json.loads(json_data)
You can now work with the data as with regular python dictionaries.
>>> print(my_dict.keys())
dict_keys(['dictionary', 'boolean', 'decimal', 'list', 'key 2', 'key 1'])
>>> print(my_dict.values())
dict_values([{'child key 1': 'child value 1', 'child key 2': 'child value 2'}, True, 10, [1, 2, 3], 'value 2', 'value 1'])
>>> print(my_dict['list'])
[1, 2, 3]
>>> print(my_dict['dictionary'])
{'child key 1': 'child value 1', 'child key 2': 'child value 2'}
>>> print(my_dict['dictionary']['child key 1'])
child value 1
You can use the dumps() function to create a JSON formatted string from a dictionary, like in the following example:
import json
my_dictionary = {
"key 1": "Value 1",
"key 2": "Value 2",
"decimal": 100,
"boolean": False,
"list": [1, 2, 3],
"dict": {
"child key 1": "value 1",
"child key 2": "value 2"
}
}
print(json.dumps(my_dictionary))
{"boolean": false, "decimal": 100, "list": [1, 2, 3], "key 2": "Value 2", "key 1": "Value 1", "dict": {"child key 1": "value 1", "child key 2": "value 2"}}
The output from this dumps command is quite complicated, because it won't use any kind of indentation by default. To create a more readable output, you can add the indent parameter to the function call, which will add some hierarchy and indention to the output.
>>> print(json.dumps(my_dictionary, indent=4))
{
"boolean": false,
"decimal": 100,
"list": [
1,
2,
3
],
"key 2": "Value 2",
"key 1": "Value 1",
"dict": {
"child key 1": "value 1",
"child key 2": "value 2"
}
}
By default, the keys within a python dictionary are unsorted and the output of the json.dumps() function may be different when executing multiple times. I ran into this issue while writing some test cases, but setting the sort_keys parameter to true will solve the problem. This will sort the key values of the dictionary and will produce always the same output when using the same data.
>>> print(json.dumps(my_dictionary, indent=4, sort_keys=True))
{
"boolean": false,
"decimal": 100,
"dict": {
"child key 1": "value 1",
"child key 2": "value 2"
},
"key 1": "Value 1",
"key 2": "Value 2",
"list": [
1,
2,
3
]
}